Shift Register: An Overview
A shift register is a set of sequential registers placed in series to transfer and store data. The figure below shows a 4-bit serial shift register.
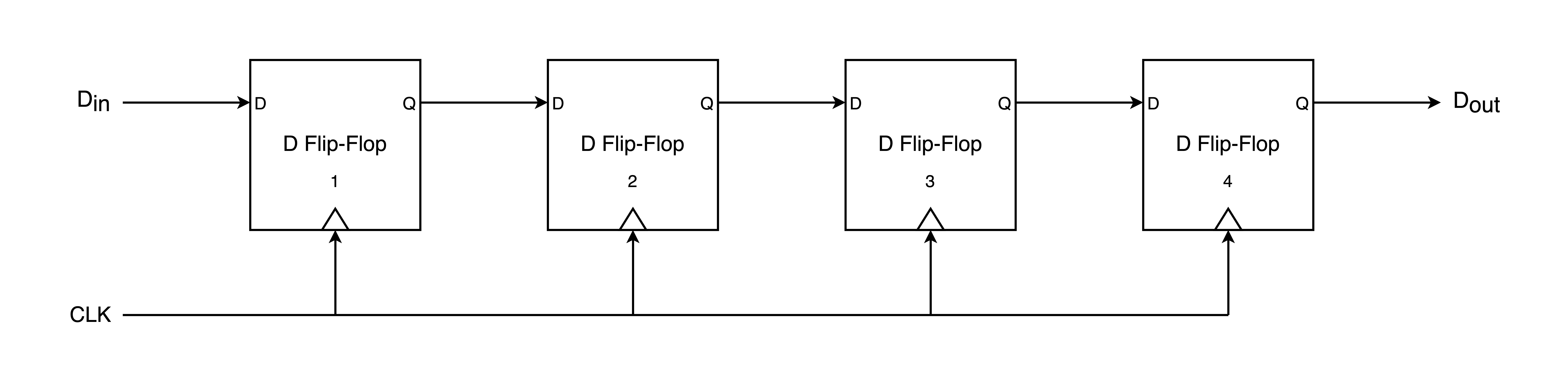
The waveform below shows the data being shifted from flip-flop 1 to 4 on the rising edge of the clock.
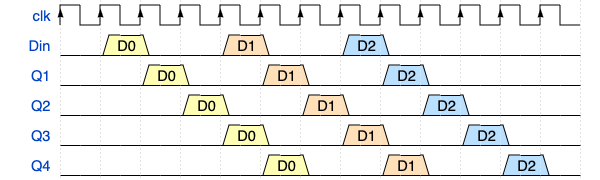
Applications
Shift registers are typically used to collect and transfer data for various applications. They are also used for data conversion, the types of data converters are listed below:
- Serial In - Serial Out (SISO)
- Serial In - Parallel Out (SIPO)
- Parallel In - Serial Out (PISO)
- Parallel In - Parallel Out (PIPO)
Used in counters for counting events or pulses.
The power of two multiplication and division is performed by simple bit-shifting operations.
Verilog Code: 4-bit Shift Register
module shift_register #(parameter N = 4)(/*AUTOARG*/
// Outputs
dout,
// Inputs
clk, rst, din
);
// Outputs
output dout;
// Inputs
input clk;
input rst;
input din;
logic [N-1:0] regs;
/*AUTOREG*/
/*AUTOWIRE*/
always_ff@(posedge clk)
if(rst)
regs <= 0;
else
regs <= {regs[N-2:0], din};
assign dout = regs[N-1];
endmodule